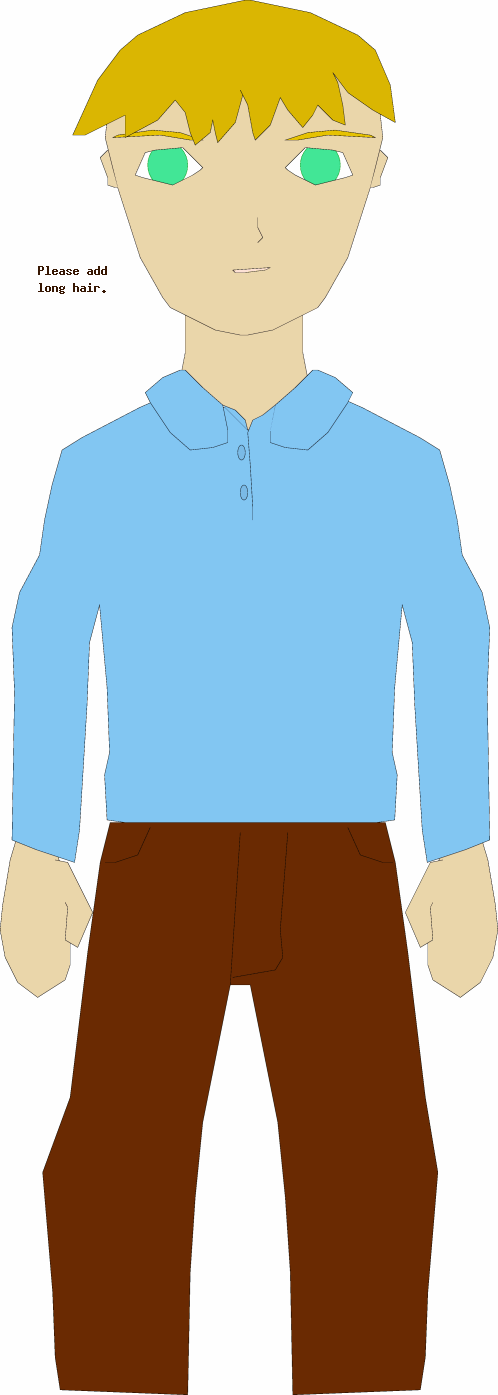
Jan 24, 2004 by Javantea
0.7 days and another MoHM hits the shelf. I'm working on Hack Mars in case you're new here. I hope today's lesson will be short because I want to work. Below is a screenshot of what Hack Mars v4.0 r0.22 looks like.
No, it's not an explosion. You've seen better SUVs in your life, haven't you? Yup, it's a bug in the new rendering software which I am writing and debugging. Below is the code that I am debugging, trying to make it work and produce a correct SUV.
if (m_iMaterials > 0 && m_pMaterials[0].texture != 0) { glEnable(GL_TEXTURE_2D); glBindTexture(GL_TEXTURE_2D, m_pMaterials[0].texture); //cout << m_pMaterials[0].texture; } else { glDisable(GL_TEXTURE_2D); } //========================// // set the array pointers // //========================// glEnableClientState(GL_VERTEX_ARRAY); glEnableClientState(GL_TEXTURE_COORD_ARRAY); //glEnableClientState(GL_NORMAL_ARRAY); //glDisableClientState(GL_WEIGHT_ARRAY_ARB); //cout << &(((litVertex *)m_pPoints)[0].position.x) << &(((litVertex *)m_pPoints)[0].position.y) << &(((litVertex *)m_pPoints)[0].position.z) << endl; glVertexPointer(3, GL_FLOAT, sizeof(litVertex), &(((litVertex *)m_pPoints)[0].position.x)); //glNormalPointer(GL_FLOAT, sizeof(litVertex), &(((litVertex *)m_pPoints)[0].normal)); glTexCoordPointer(2, GL_FLOAT, sizeof(litVertex), &(((litVertex *)m_pPoints)[0].tu0)); //cout << "b " << pSurfaces[0].m_vCorners[0] << " " << pSurfaces[0].m_vCorners[1] << " " << pSurfaces[0].m_vCorners[2] << endl; // Find the list of triangles owned by this group. face *pMyTris; //if(m_pTriangles[0] + m_iTriangles) pMyTris = &(m_pSurfaces[0]); //else // pMyTris = &(pSurfaces[0]); //======================// // render the triangles // //======================// glDrawElements(GL_TRIANGLES, m_iSurfaces * 3, GL_UNSIGNED_SHORT, pMyTris); // GL_UNSIGNED_INTObviously, there's more code that holds vertex data, triangle data, and texture date. My lesson for today is that of step by step debugging. That is the most useful thing that I have as a programmer besides fast demo prototyping. If a programmer has both skills, they are a mad machine on the loose trying to digitize the world. Demo prototyping is a system where instead of writing one large program that has many parts, you write many little programs that do their own thing. When you want one program out of them, you just merge all the classes together. Let's start by analyzing the pros and cons of fast demo prototyping.
Pros
Demos are easy.
Testing is quick.
It looks like you're doing more work than you are.
One person can get the job done in less than one day.
There's always a working backup.
Cons
If the demo doesn't work, you're in trouble.
The integration of the demo can cause problems.
If you don't get it right the first time, usually you must back to the drawing board.
Complex systems requiring heavyweight external libraries are impossible to demo.
Debugging is sometimes ugly.*
* This is why I need step by step debugging.
So you can see that most of the problems with fast demo prototyping are caused by a few ugly lines of code. Well, there's a system I came up with called step by step debugging. It solves the problem when one bug that will not do right. I have that problem right now and I'll show you how it works by solving that problem.
- The problem involves the rendering.
- The rendering involves indices and vertices.
- The vertices and indices involve loading from a data file.
- The data file is created from another data file.
- The first data is created by Milkshape3D.
- The rendering is assumed to work. Evidence is clear.
- The indices and vertices are not working at the rendering level.
- The loading of vertices from the data file is working. The printout below shows clearly that vertices are being loaded correctly.
p-19.975 30.4093 30.4093; -19.975 30.4093 65.9007; p-27.1937 26.8455 26.8455; -27.1937 26.8455 67.259; p 17.1886 30.4093 30.4093; 17.1886 30.4093 65.9007; p 24.4072 26.8455 26.8455; 24.4072 26.8455 67.259; p 23.8913 41.3856 41.3856; 23.8913 41.3856 48.2109; p 28.4678 26.1456 26.1456; 28.4678 26.1456 53.9481; p 22.3764 45.7618 45.7618; 22.3764 45.7618 9.84992;
The loading of indices are known to work. Yet another printout to ensure that. Except that the printout tells us the problem.i 0 0 1; 0 0 1; i 1 0 3; 1 0 3; i 4 0 5; 4 0 5; i 5 0 7; 5 0 7; i 8 0 9; 8 0 9;
- The data file is created from another data file. We know due to the above printout that it is not working.
- The first data file is good. It has been tested with Milkshape3D and HM 4.21.
Some programmers might say: "But I write well commented, high quality code slowly. No demoing for me!" I say that these programmers are wasting time. Demos ensure that code is being written all the time. The fact that the demo works after the second function has been written means that the quality is always ensured. While a programmer might be lulled into writing code that works but is not quality by the demo system, I believe that any programmer that is motivated to write good quality code and has the skills to do it can write good quality code, commented or not.
The only problem with uncommented code is that you are the sole owner of it. But imagine a world where you lack peers. No one can add to your code anyway. It's better to let the code walk than talk. Writing descriptions of functions is nice, but it is redundant if your code is self-commenting. A function called render can do just one thing: render. A variable called vertices can be only one thing: position data.
I hope you have learned a little and seen my philosophy of coding. If it screams Visual Basic 6.0, then wait until I release HM and then criticize the code.

-
Leave a Reply
Comments: 0
Leave a reply »